Introducing Typeconf
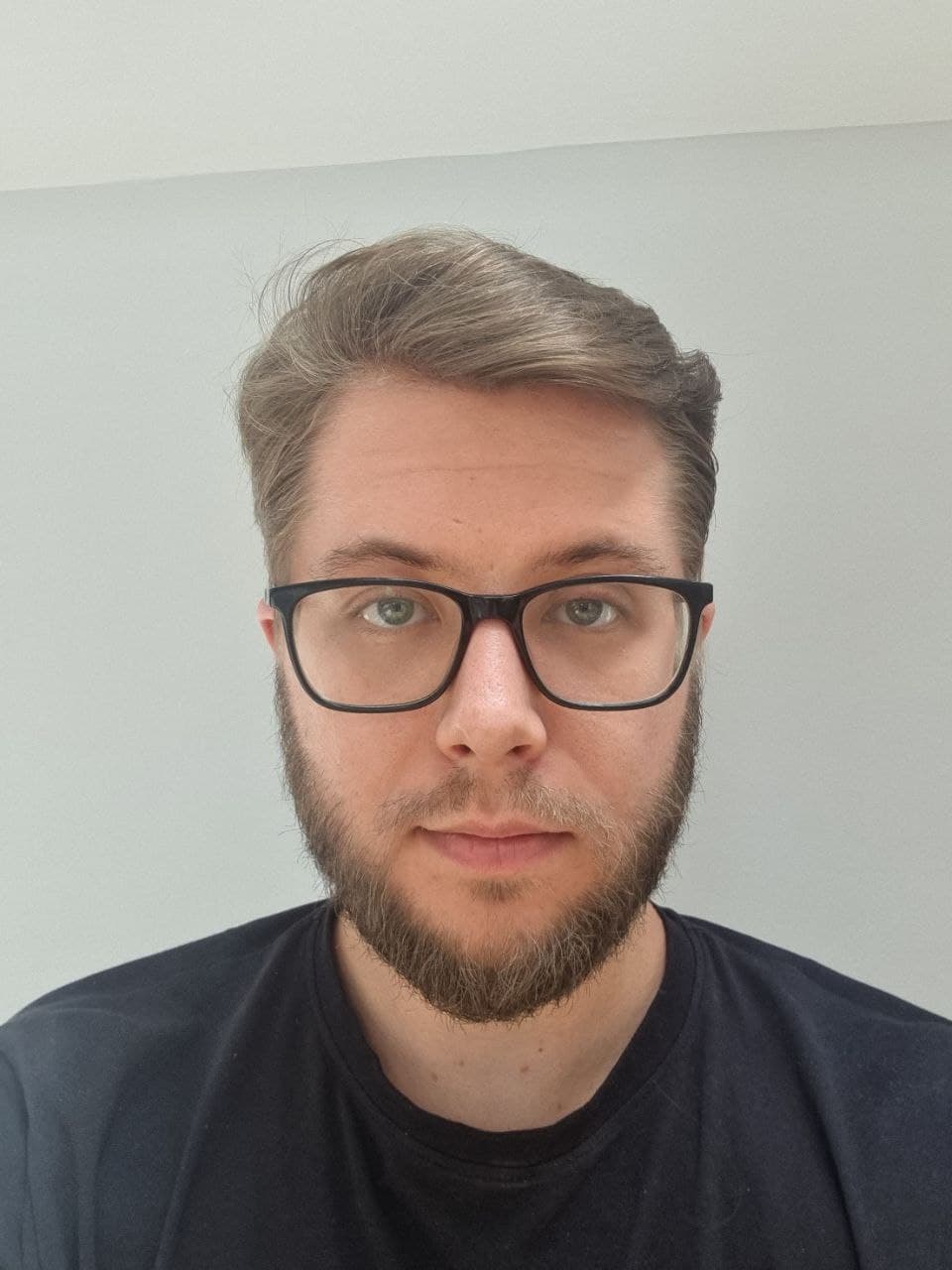
Hey there! 👋 I'm excited to share something we've been working on. It's called Typeconf, and it's a new way to handle configuration in your apps using TypeScript and TypeSpec.
The Problem
We've all been there. You're building an app, and your config files start getting out of hand:
api:
endpoints:
users:
rate_limit: 1000 # is this per second? per minute?
timeout: 30 # seconds? milliseconds?
retries: 3
YAML and JSON are great, but they have some serious limitations:
- No type safety (hope you like runtime errors!)
- Zero autocomplete in your IDE
- No way to share types between configs
- Comments are your only documentation
Enter Typeconf
Typeconf lets you write your configuration like this:
model APIConfig {
@doc("Rate limit per minute")
rate_limit: int32;
@doc("Timeout in milliseconds")
timeout: Duration;
@doc("Number of retry attempts")
retries: int32;
}
// Your actual config
let config: APIConfig = {
rate_limit: 1000,
timeout: "30s",
retries: 3
};
Now you get:
- ✨ Full type safety
- 🚀 Amazing IDE support
- 📚 Built-in documentation
- 🔄 Type sharing between services
Real World Use Cases
Here are some ways people are already using Typeconf:
1. Feature Flags
model FeatureFlag {
enabled: boolean;
rollout_percentage: float32;
target_users: string[];
}
let features: Record<FeatureFlag> = {
"dark_mode": {
enabled: true,
rollout_percentage: 50.0,
target_users: ["beta_testers"]
}
};
2. Infrastructure Config
model ServiceConfig {
replicas: int32;
memory_limit: string;
environment: Record<string>;
}
let services = {
"api": {
replicas: 3,
memory_limit: "512Mi",
environment: {
NODE_ENV: "production"
}
}
};
Getting Started
It's super easy to try Typeconf:
npx create-typeconf-package my-config
cd my-config
npm run dev
What's Next?
This is just the beginning. Here's what's coming:
- 🔒 Schema validation in reading API
- 💾 Network storage
- 🌐 Multi-language support
- 🔄 Schema conversion: Read configs from YAML, TOML, JSON, and more
Join the Community
Typeconf is open source and we'd love your help! Here's how you can get involved:
Try It Now
Want to see it in action? Check out our playground or dive into the docs.
Let me know what you think! I'm @ichebykin on Twitter - I'd love to hear your feedback and ideas.
P.S. If you found this interesting, consider giving us a ⭐ on GitHub. It really helps!