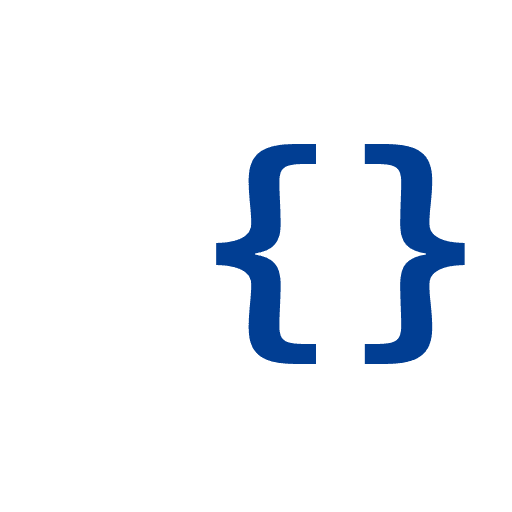
Update system prompts without redeployment
Imagine writing product configs as code
Typeconf is an open-source platform for writing live configs as code.
Create your project and save time on deployment small changes with type-safe configs and get realtime updates.
Features
Type-Safe Configuration Database
- Full IDE (Cursor, Windsurf, VSCode) support
- Compile-time type checking
- Deploy any configuration: feature flags, A/B tests, LLM configs
Runtime Schema Validation
Made by builders, for builders
NPM Integration
Multi-Platform Support
AI Ready
Coming Soon: Secrets
Use Cases
Product Configuration
Feature flags, user settings, and product parameters
Quickly iterate on your LLM prompts
model AgentConfig {
prompt: string;
temperature: int32;
maxTokens: int32;
searchDepth: int32;
searchUrl: string;
}
let agentConfig: AgentConfig = {
prompt: `You're an assistant
that searches the web to
improve configurations
of software across the world.`,
temperature: 0.5,
maxTokens: 10000,
searchDepth: 10,
};
Service Configuration
API endpoints, business rules, and service settings
Define and manage API endpoints with type safety
model APIConfig {
endpoints: Record<{
url: string;
method: "GET" | "POST";
timeout: int32;
retries: int32;
}>;
rateLimiting: Record<{
requests: int32;
window: string;
}>;
}
let api: APIConfig = {
endpoints: {
"users": {
url: "/api/v1/users",
method: "GET",
timeout: 5000,
retries: 3
}
},
rateLimiting: {
"default": {
requests: 100,
window: "1m"
}
}
};
Infrastructure Configuration
Deployment settings, networking, and infrastructure parameters
Manage infrastructure and deployment settings
model Deployment {
replicas: int32;
resources: Record<string>;
environment: "dev" | "prod";
scaling: Record<{
min: int32;
max: int32;
target: int32;
}>;
}
let deployment: Deployment = {
replicas: 3,
resources: {
"cpu": "2",
"memory": "4Gi"
},
environment: "prod",
scaling: {
"default": {
min: 2,
max: 10,
target: 5
}
}
};
Loved by Developers
Looks very interesting, will give it a go. What are the other languages you plan to support and what would be the use cases of shared types between languages? This might be super powerful in microservices architecture, have you thought about it?
This seems interesting, but how can I use it in projects that aren't using TS/JS?
Looks great! I have to ask tho: is the plan with the "free cloud storage" for configs to eventually start charging?
Thanks for your info, I’ll look into it! So it is a safe way to toggle feature flags, right?
Def want to start integrating typeconf for feature flags, cuz i want some users to see the reddit montioring feature, and others just to see the linkedin posting feature
Looks very interesting, will give it a go. What are the other languages you plan to support and what would be the use cases of shared types between languages? This might be super powerful in microservices architecture, have you thought about it?
This seems interesting, but how can I use it in projects that aren't using TS/JS?
Looks great! I have to ask tho: is the plan with the "free cloud storage" for configs to eventually start charging?
Thanks for your info, I’ll look into it! So it is a safe way to toggle feature flags, right?
Def want to start integrating typeconf for feature flags, cuz i want some users to see the reddit montioring feature, and others just to see the linkedin posting feature
Will: someone invent ai infra so i can yell at aws and have impact me: what kind of infra do you want? :) Will: one that uses typeconf! and honestly it would be pretty cool if there was an agent that could provision your cloud stack by analyzing your code
Wow I’m curious how to use it. I want to use it for feature flagging. I keep adding UUIDS to my GitHub code and then pushing it live. Sometimes I’m in the middle of coding something and it’s horrible having to rebuild, rollback, if there’s a bug…. Much rather be able to update a config. For my marketing copy or uuids to test features…
I’ll check it out
me: I feel like I should just be able to generate k8s configs from Typescript with Typeconf if I add all the types there, is anyone interested in this? sudosteph: Yes. CDK has made me a fan of Typescript.
Will: someone invent ai infra so i can yell at aws and have impact me: what kind of infra do you want? :) Will: one that uses typeconf! and honestly it would be pretty cool if there was an agent that could provision your cloud stack by analyzing your code
Wow I’m curious how to use it. I want to use it for feature flagging. I keep adding UUIDS to my GitHub code and then pushing it live. Sometimes I’m in the middle of coding something and it’s horrible having to rebuild, rollback, if there’s a bug…. Much rather be able to update a config. For my marketing copy or uuids to test features…
I’ll check it out
me: I feel like I should just be able to generate k8s configs from Typescript with Typeconf if I add all the types there, is anyone interested in this? sudosteph: Yes. CDK has made me a fan of Typescript.