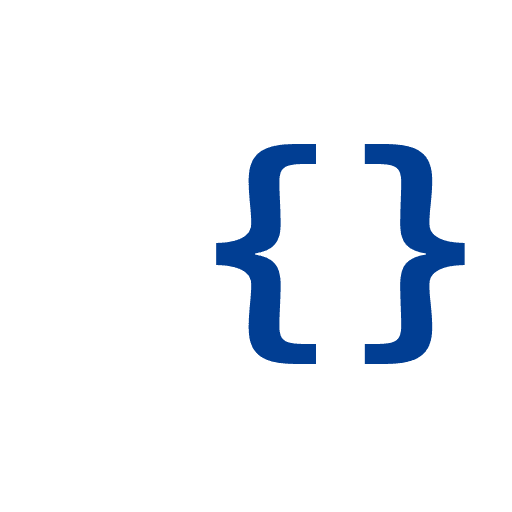
Typeconf
Adding types for your configuration
npm install -g @typeconf/typeconf
Describe config schema
model ProjectConfig {
name: string;
modes: Array<string>;
pricingCoeffs: Record<float32>;
}
Add your config values with types
import {
ProjectConfig,
} from "@root/types/all.js";
let config: ProjectConfig = {
name: "my-service",
modes: ["current", "new_test"],
pricingCoeffs: {
"london": 0.99,
"nyc": 1.25,
"sf": 9000.0,
},
};
export default config;
Get your config with types
// read config in your code
import {ProjectConfig} from "@/typeconf-gen/all";
import {readConfigFromFile} from "@typeconf/sdk";
let projectConfig: ProjectConfig =
readConfigFromFile("configs-dir/out/name.json");
Or use the produced JSON
{
"name": "my-service",
"availableModes": [
"current",
"new_test"
],
"pricingCoeffs": {
"london": 0.99,
"nyc": 1.25,
"sf": 9000.0
}
}
Configuration is messy. We have many different formats, YAML, JSON, random ini files, not to mention environment variables. It's hard to track changes and easy to break stuff. So we thought let's add types there! Our tool, Typeconf, allows you to define types for your configuration and read it in your code.
Features
Write configuration using Typescript and never worry about type issues.
Easily share configs between projects using just NPM.
Define configuration types using the TypeSpec format with VSCode support.
Read configs from your code into first class types. Currently we support Typescript.
Use Cases
model ProductParams { deviceType: string; coefficient: number; isEnabled: boolean; } model ProductFlags { params_by_user_id: Record<ProductParams>; params_by_device: Record<ProductParams>; }
Feature flags and gradual rollouts
With Typeconf you can define product flags based on device type, user id, and whatever else you want.
Infrastructure configs
You can replace tons of hard to support YAMLs with simple configuration in Typescript.
// types model Route { path: string; backend: string; rateLimit: int32; } model APIGatewayConfig { routes: Record<Route>; } // values import { APIGatewayConfig } from "@root/types/all.js"; let config: APIGatewayConfig = { routes: { "/user": { path: "/user", backend: "user-service", rateLimit: 1000, }, "/order": { path: "/order", backend: "order-service", rateLimit: 500, }, }, }; export default config;
model Employee { employeeId: string; name: string; email: string; role: string; } model EmployeeInventory { employees: Record<Employee>; }
Inventory storage
You can define Typeconf configs for your data that changes rarely, like employee portal information, device firmware list.